dotunit is a port of
JUnit (www.junit.org) to the Microsoft .net
platform. It provides a testing framework to support automated testing of
applications, particularly to facilitate unit, function and regression testing.
2.
Automated Testing
Testing is tedious and time
consuming. As a result, it is not a popular chore among developers and few do it
thoroughly, if at all. However, software must be tested to maintain quality and
stability.
More often than not, a
developer’s code does not work the first time (or the second, third…). The
debugging process is often interactive and iterative. This requires the repeated
testing of the same code.
The cost of testing does not
stop with initial debugging unfortunately. As the system develops and is
revised, regression testing is necessary to ensure previous sections still
function properly as new ones are added.
Automated testing frameworks
provide the developer with a method of capturing test cases and automate their
execution, validation and the collection of results. The cost of testing goes
down considerably and the developer can grow in confidence that the quality and
stability of his code is high, without the excessive cost of traditional
testing.
(For more on the value of
automated testing, please see http://junit.sourceforge.net/doc/testinfected/testing.htm )
3.
Getting Started
·
After installing dotunit, open the project you
wish to test in the Visual Studio.net IDE.
·
Add a reference to dotunit.framework.dll (You may
need to browse to locate the dll)
In general, tests are methods in a class
that descends from dotUnit.Framework.TestCase. Each method that begins
with the word Test (case matters) is a test case.
Such functions take no arguments and should not return anything.
Each value that should be
tested is done with either:
void AssertTrue(bool expression)
void
AssertEquals(…)
(AssertEquals is overloaded for each
simple data type (bool, string, int, double, etc.) The general format is
(optional string
message, expected value, actual value)
4.
Simple Tests
The
following is a simple test case.
public class
SimpleTest : dotUnit.Framework.TestCase
{
public void
TestSimpleCase()
{
AssertEquals(5,
2+3);
}
}
5.
Fixtures
Fixtures are
contexts for a series of tests. Many tests will use a common set of
objects/state for testing. A fixture provides this common context. In order to
establish a fixture, override the SetUp
and TearDown (optional)
methods. SetUp will be called before any tests
are run and TearDown will be
called after the tests are run.
public class SimpleFixture:
dotUnit.Framework.TestCase
{
private
m_nTestValue;
protected
override void SetUp()
{
m_nTestValue =
5;
}
public void TestSimpleCase()
{
AssertEquals(m_nTestValue, 2+3);
}
}
6.
Suites
Test Suites
are collections of tests. You can arrange a hierarchy of test cases, as suites
can contain other suites or test cases. Suites organize your tests.
In order to
define a suite, create a class that implements a Suite property (return a
TestSuite).
public class MySuite:
{
public
dotUnit.Framework.TestSuite Suite
{
get
{
dotUnit.Framework.TestSuite
suite = new
dotUnit.Framework.TestSuite(“My
Tests”);
suite.AddTestSuite(typeof(SimpleTest));
suite.AddTestSuite(typeof(SimpleFixture));
}
}
}
7.
Running Tests
The
dotunit GUI can be used to run your tests.
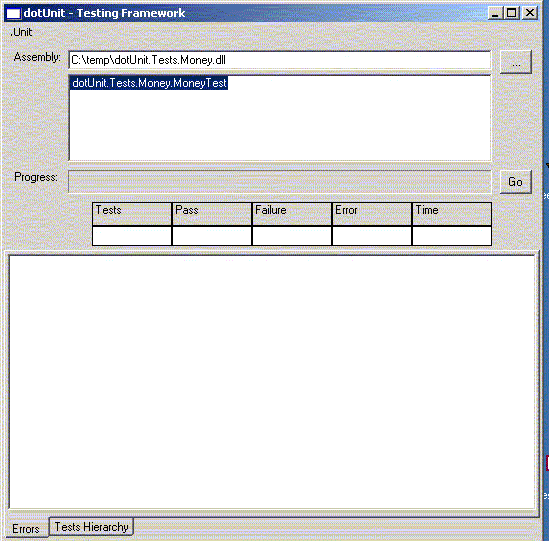
Simply
browse to you compiled test cases (dll), select the class to test and click
Go. You will see the results in the Errors window. You can browse
the test hierarchy as well.
8.
Running Tests from within a Test
Project
Set a reference to
dotUnit.GUI. Assuming your test project is a console application, use the
following Main definition:
static void Main(string[]
args)
{
dotUnit.GUI.GUIRunner.Run(new MyTestCase("TestSomething"));
}
In the above, MyTestCase is the TestCase and “TestSomething” is the
name of the test routine to run. If there are multiple tests/cases, it is
advisable to use a TestSuite
instead of a TestCase
(instead of MyTestCase).
9.
Testing Internal / Private Classes and
Methods
Quite often,
it is useful to unit test internal and private methods, that support the
implementation of larger functionality. It is time consuming and error-prone to
toggle access modifiers between “public” and “private”.
dotunit provides several utility functions to allow for the
testing of non-public methods and classes. The following code snippet highlights
the functions. (Please see the documentation for dotUnit.Utility.NonPublicType and the
dotUnit.Tests.NonPublicTypeTest
for more information.)
public void
TestMethods()
{
Type[] a =
{typeof(int)};
object[]
aValues={TEST_VALUE};
AssertEquals(TEST_VALUE+TEST_VALUE,
(int)NonPublicType.InvokeFunction(m_target,
"ProtectedAdd", a,
aValues));
}
(In the
above example, m_target is a reference to an object that has non-public
methods.)
|